vbty
Monday, March 15, 2021
ACCESS_MASK: Specific rights of NT objects
#define FILE_READ_DATA ( 0x0001 ) // file & pipe
#define FILE_LIST_DIRECTORY ( 0x0001 ) // directory
#define FILE_WRITE_DATA ( 0x0002 ) // file & pipe
#define FILE_ADD_FILE ( 0x0002 ) // directory
#define FILE_APPEND_DATA ( 0x0004 ) // file
#define FILE_ADD_SUBDIRECTORY ( 0x0004 ) // directory
#define FILE_CREATE_PIPE_INSTANCE ( 0x0004 ) // named pipe
#define FILE_READ_EA ( 0x0008 ) // file & directory
#define FILE_WRITE_EA ( 0x0010 ) // file & directory
#define FILE_EXECUTE ( 0x0020 ) // file
#define FILE_TRAVERSE ( 0x0020 ) // directory
#define FILE_DELETE_CHILD ( 0x0040 ) // directory
#define FILE_READ_ATTRIBUTES ( 0x0080 ) // all
#define FILE_WRITE_ATTRIBUTES ( 0x0100 ) // all
#define FILE_ALL_ACCESS (STANDARD_RIGHTS_REQUIRED | SYNCHRONIZE | 0x1FF)
#define FILE_GENERIC_READ (STANDARD_RIGHTS_READ |\
FILE_READ_DATA |\
FILE_READ_ATTRIBUTES |\
FILE_READ_EA |\
SYNCHRONIZE)
#define FILE_GENERIC_WRITE (STANDARD_RIGHTS_WRITE |\
FILE_WRITE_DATA |\
FILE_WRITE_ATTRIBUTES |\
FILE_WRITE_EA |\
FILE_APPEND_DATA |\
SYNCHRONIZE)
#define FILE_GENERIC_EXECUTE (STANDARD_RIGHTS_EXECUTE |\
FILE_READ_ATTRIBUTES |\
FILE_EXECUTE |\
SYNCHRONIZE)
#define PROCESS_TERMINATE (0x0001)
#define PROCESS_CREATE_THREAD (0x0002)
#define PROCESS_SET_SESSIONID (0x0004)
#define PROCESS_VM_OPERATION (0x0008)
#define PROCESS_VM_READ (0x0010)
#define PROCESS_VM_WRITE (0x0020)
#define PROCESS_DUP_HANDLE (0x0040)
#define PROCESS_CREATE_PROCESS (0x0080)
#define PROCESS_SET_QUOTA (0x0100)
#define PROCESS_SET_INFORMATION (0x0200)
#define PROCESS_QUERY_INFORMATION (0x0400)
#define PROCESS_SUSPEND_RESUME (0x0800)
#define PROCESS_QUERY_LIMITED_INFORMATION (0x1000)
#define PROCESS_SET_LIMITED_INFORMATION (0x2000)
#define PROCESS_ALL_ACCESS (STANDARD_RIGHTS_REQUIRED | SYNCHRONIZE | \
0xFFFF)
#define THREAD_TERMINATE (0x0001)
#define THREAD_SUSPEND_RESUME (0x0002)
#define THREAD_GET_CONTEXT (0x0008)
#define THREAD_SET_CONTEXT (0x0010)
#define THREAD_QUERY_INFORMATION (0x0040)
#define THREAD_SET_INFORMATION (0x0020)
#define THREAD_SET_THREAD_TOKEN (0x0080)
#define THREAD_IMPERSONATE (0x0100)
#define THREAD_DIRECT_IMPERSONATION (0x0200)
#define THREAD_SET_LIMITED_INFORMATION (0x0400) // winnt
#define THREAD_QUERY_LIMITED_INFORMATION (0x0800) // winnt
#define THREAD_RESUME (0x1000) // winnt
#if (NTDDI_VERSION >= NTDDI_VISTA)
#define THREAD_ALL_ACCESS (STANDARD_RIGHTS_REQUIRED | SYNCHRONIZE | \
0xFFFF)
#define JOB_OBJECT_ASSIGN_PROCESS (0x0001)
#define JOB_OBJECT_SET_ATTRIBUTES (0x0002)
#define JOB_OBJECT_QUERY (0x0004)
#define JOB_OBJECT_TERMINATE (0x0008)
#define JOB_OBJECT_SET_SECURITY_ATTRIBUTES (0x0010)
#define JOB_OBJECT_IMPERSONATE (0x0020)
#define JOB_OBJECT_ALL_ACCESS (STANDARD_RIGHTS_REQUIRED | SYNCHRONIZE | \
0x3F )
Wednesday, November 13, 2019
Process Mitigations in 2019 (1)
In the recent years,Microsoft has add many mitigations in the Windows system. In this article i will show you the implementation of these mitigations.
The first question is how many mitigations have been added in Windows and what they are.
If you have even used a process manager tool which named Process Hacker, you can query each process's mitigation status.For example:
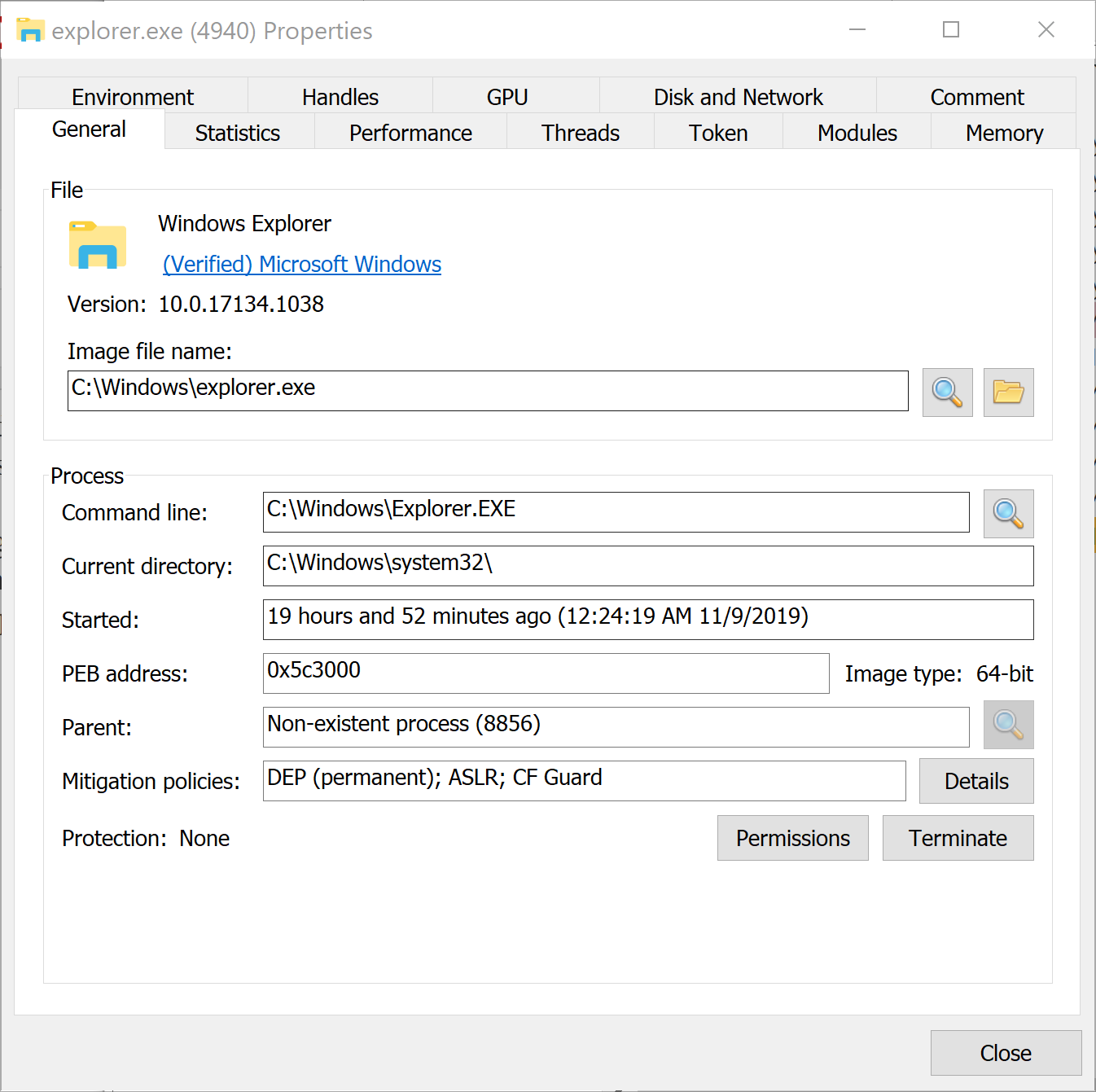
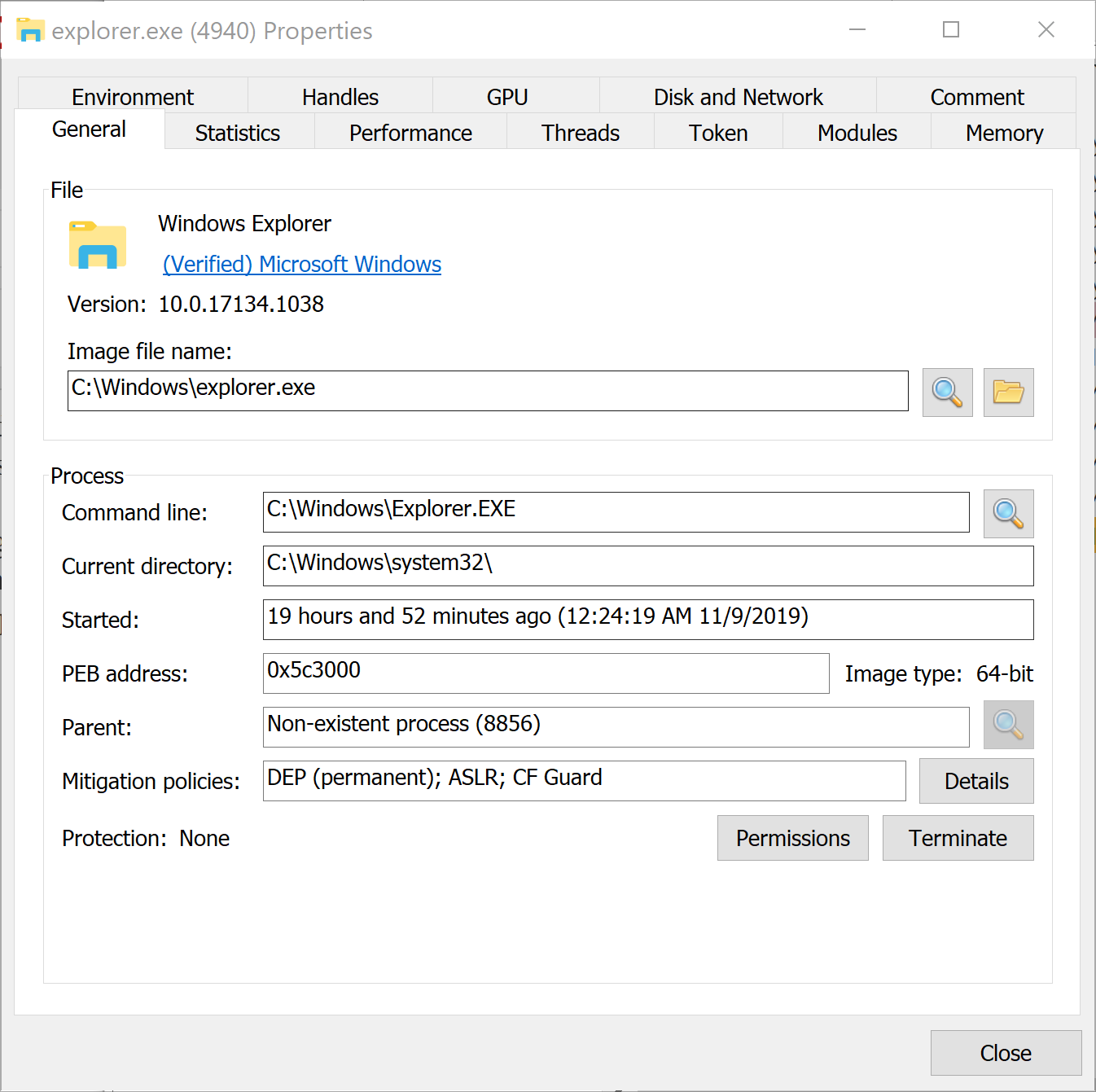
In fact, there is a struct named PROCESS_MITIGATION_POLICY in the SDK header file.
typedef enum _PROCESS_MITIGATION_POLICY {
ProcessDEPPolicy,
ProcessASLRPolicy,
ProcessDynamicCodePolicy,
ProcessStrictHandleCheckPolicy,
ProcessSystemCallDisablePolicy,
ProcessMitigationOptionsMask,
ProcessExtensionPointDisablePolicy,
ProcessControlFlowGuardPolicy,
ProcessSignaturePolicy,
ProcessFontDisablePolicy,
ProcessImageLoadPolicy,
ProcessSystemCallFilterPolicy,
ProcessPayloadRestrictionPolicy,
ProcessChildProcessPolicy,
ProcessSideChannelIsolationPolicy,
MaxProcessMitigationPolicy
} PROCESS_MITIGATION_POLICY, *PPROCESS_MITIGATION_POLICY;
If you want to know the mitigation status of a process, you can use the Windows Native API NtQueryInformationProcess.
NtQueryInformationProcess declaration is following:
NtQueryInformationProcess declaration is following:
NTSYSCALLAPI
NTSTATUS
NTAPI
NtQueryInformationProcess(
_In_ HANDLE ProcessHandle,
_In_ PROCESSINFOCLASS ProcessInformationClass,
_Out_writes_bytes_(ProcessInformationLength) PVOID ProcessInformation,
_In_ ULONG ProcessInformationLength,
_Out_opt_ PULONG ReturnLength
);
ProcessInformationClass is an enumeration that indicate the function code. ProcessMitigationPolicy(52) is used for querying the process mitigation policy. When you assign ProcessInformationClass argument as ProcessMitigationPolicy,the ProcessInformationLength is a pointer to a PROCESS_MITIGATION_POLICY_INFORMATION.
typedef struct _PROCESS_MITIGATION_POLICY_INFORMATION
{
PROCESS_MITIGATION_POLICY Policy;
union
{
PROCESS_MITIGATION_ASLR_POLICY ASLRPolicy;
PROCESS_MITIGATION_STRICT_HANDLE_CHECK_POLICY StrictHandleCheckPolicy;
PROCESS_MITIGATION_SYSTEM_CALL_DISABLE_POLICY SystemCallDisablePolicy;
PROCESS_MITIGATION_EXTENSION_POINT_DISABLE_POLICY ExtensionPointDisablePolicy;
PROCESS_MITIGATION_DYNAMIC_CODE_POLICY DynamicCodePolicy;
PROCESS_MITIGATION_CONTROL_FLOW_GUARD_POLICY ControlFlowGuardPolicy;
PROCESS_MITIGATION_BINARY_SIGNATURE_POLICY SignaturePolicy;
PROCESS_MITIGATION_FONT_DISABLE_POLICY FontDisablePolicy;
PROCESS_MITIGATION_IMAGE_LOAD_POLICY ImageLoadPolicy;
PROCESS_MITIGATION_SYSTEM_CALL_FILTER_POLICY SystemCallFilterPolicy;
PROCESS_MITIGATION_PAYLOAD_RESTRICTION_POLICY PayloadRestrictionPolicy;
PROCESS_MITIGATION_CHILD_PROCESS_POLICY ChildProcessPolicy;
PROCESS_MITIGATION_SIDE_CHANNEL_ISOLATION_POLICY SideChannelIsolationPolicy;
};
} PROCESS_MITIGATION_POLICY_INFORMATION, *PPROCESS_MITIGATION_POLICY_INFORMATION;
This is a peach of NtQueryInformationProcess disassembly code in the ntoskrnl.By the reserve enginning we found that we must have the PROCESS_QUERY_INFORMATION permission with the process handle to invoke this function.
case 52: // ProcessMitigationPolicy
if (ProcessInformationLength != 8 )
return 0xC0000004;
MitigationCode = *(_DWORD *)ProcessInformation;
if ( Handle == (HANDLE)-1i64 )
{
pObject = (PEPROCESS)PsGetCurrentProcess();
}
else
{
result = ObReferenceObjectByHandleWithTag(
(ULONG_PTR)Handle,
0x400, //PROCESS_QUERY_INFORMATION
PsProcessType,
PreviousMode,
'yQsP',
(__int64)&pObject,
0i64);
if ( result < 0 )
return result;
}
So we can write a simple progarm to query the mitigation status of a process. It look like this:
ClientId.UniqueProcess = PID;
NtOpenProcess(
&ProcHandle,
PROCESS_QUERY_INFORMATION,
&ObjAttr,
&ClientId);
NtQueryInformationProcess(
ProcHandle,
ProcessMitigationPolicy,
RecvBuffer,
RecvBufferSize,
&RetSize);
Then i query the Microsoft Edge content process by this tool, and get the mitigation policy information as below.
[*]ProcessDEPPolicy:
MEM_EXECUTE_OPTION_DISABLE 1
MEM_EXECUTE_OPTION_ENABLE 0
MEM_EXECUTE_OPTION_DISABLE_THUNK_EMULATION 1
MEM_EXECUTE_OPTION_PERMANENT 1
MEM_EXECUTE_OPTION_EXECUTE_DISPATCH_ENABLE 0
MEM_EXECUTE_OPTION_IMAGE_DISPATCH_ENABLE 0
MEM_EXECUTE_OPTION_VALID_FLAGS 1
[*]ProcessASLRPolicy:
EnableBottomUpRandomization 1
EnableForceRelocateImages 1
EnableHighEntropy 1
DisallowStrippedImages 1
[*]ProcessDynamicCodePolicy:
ProhibitDynamicCode 0
AllowThreadOptOut 0
AllowRemoteDowngrade 0
AuditProhibitDynamicCode 0
[*]StrictHandleCheckPolicy:
RaiseExceptionOnInvalidHandleReference 1
HandleExceptionsPermanentlyEnabled 1
[*]SystemCallDisablePolicy:
DisallowWin32kSystemCalls 0
AuditDisallowWin32kSystemCalls 0
ProcessExtensionPointDisablePolicy:
DisableExtensionPoints 0
[*]ProcessControlFlowGuardPolicy:
EnableControlFlowGuard 1
EnableExportSuppression 1
StrictMode 0
[*]ProcessSignaturePolicy:
MicrosoftSignedOnly 0
StoreSignedOnly 0
MitigationOptIn 0
AuditMicrosoftSignedOnly 0
AuditStoreSignedOnly 0
[*]ProcessFontDisablePolicy:
DisableNonSystemFonts 0
AuditNonSystemFontLoading 0
[*]ProcessImageLoadPolicy:
NoRemoteImages 1
NoLowMandatoryLabelImages 1
PreferSystem32Images 0
AuditNoRemoteImages 0
AuditNoLowMandatoryLabelImages 0
[*]SystemCallFilterPolicy:
FilterId 0
[*]PayloadRestrictionPolicy:
EnableExportAddressFilter 0
AuditExportAddressFilter 0
EnableExportAddressFilterPlus 0
AuditExportAddressFilterPlus 0
EnableImportAddressFilter 0
AuditImportAddressFilter 0
EnableRopStackPivot 0
AuditRopStackPivot 0
EnableRopCallerCheck 0
AuditRopCallerCheck 0
EnableRopSimExec 0
AuditRopSimExec 0
[*]ProcessChildProcessPolicy:
NoChildProcessCreation 1
AuditNoChildProcessCreation 0
AllowSecureProcessCreation 0
[*]ProcessSideChannelIsolationPolicy:
SmtBranchTargetIsolation 0
IsolateSecurityDomain 0
DisablePageCombine 0
SpeculativeStoreBypassDisable 0
ProcessImageLoadPolicy
When get or set ProcessImageLoadPolicy mitigation by PROCESS_MITIGATION_IMAGE_LOAD_POLICY struct. And this struct's declaration is below.We can see there are 5 levels restricted for image loading.
typedef struct _PROCESS_MITIGATION_IMAGE_LOAD_POLICY {
union {
DWORD Flags;
struct {
DWORD NoRemoteImages : 1;
DWORD NoLowMandatoryLabelImages : 1;
DWORD PreferSystem32Images : 1;
DWORD AuditNoRemoteImages : 1;
DWORD AuditNoLowMandatoryLabelImages : 1;
DWORD ReservedFlags : 27;
} DUMMYSTRUCTNAME;
} DUMMYUNIONNAME;
} PROCESS_MITIGATION_IMAGE_LOAD_POLICY, *PPROCESS_MITIGATION_IMAGE_LOAD_POLICY;
In the Windows kernel section has three types.
PhysicalSection
ImageSection
DataSection
NtCreateSection is the interface to create a section object in Windows.If we specify SEC_IMAGE flag when invoke NtCreateSection ,it will set a bit flag named MMSECTION_FLAGS in section.ControlArea after the section object has be created. Then it will execute into the MiMapViewOfImageSection when we call the NtMapViewOfSection function with this section handle.
1.NtMapViewOfSection
2.MiMapViewOfSection
3.MiMapViewOfImageSection
4.MiAllowImageMap
The ProcessImageLoadPolicy mitigation is implement in the MiAllowImageMap function.
MiAllowImageMap is splice into two piece.
Firstly, it check for remote image load.
Firstly, it check for remote image load.
IsProhibitRemoteImageMapEnable = Process->MitigationFlags & 0x80000; // ProhibitRemoteImageMap
IsAuditProhibitRemoteImageMap = Process->MitigationFlags & 0x100000; // AuditProhibitRemoteImageMap
if ((IsProhibitRemoteImageMapEnable || IsAuditProhibitRemoteImageMap) &&
(Section->u1.ControlArea & 3)) // AuditProhibitRemoteImageMap
{
if ( IsProhibitRemoteImageMapEnable )
return STATUS_ACCESS_DENIED;
}
Secondly, it checks for integrity level. It checks the source image file's integrity level by the image file's file object's security descripter.
MitigationFlags = Process->MitigationFlags;
IsAuditProhibitLowILImageMap = MitigationFlags & 0x400000; // AuditProhibitLowILImageMap
IsProhibitLowILImageMap = MitigationFlags & 0x200000; // ProhibitLowILImageMap
if ( IsProhibitLowILImageMap || IsAuditProhibitLowILImageMap )
{
MemoryAllocated = 0;
FileObject = MiReferenceControlAreaFile(pControlArea);
status = ObpGetObjectSecurity(FileObject, &SecurityDescriptor, &MemoryAllocated);
if (!NT_SUCCESS(status))
{
status = STATUS_ACCESS_DENIED;
}
else
{
if (SeQueryMandatoryLabel(SecurityDescriptor) <= 0x1000 &&
!SeGetTrustLabelAce(SecurityDescriptor) )
status = STATUS_ACCESS_DENIED;
ObReleaseObjectSecurity(SecurityDescriptor, MemoryAllocated);
}
MiDereferenceControlAreaFile(pControlArea, FileObject);
}
SeQueryMandatoryLabel is a function for getting the integrity level, it's return value indicates the integrity level value.This is because of the mandatory label is a series of SID which have the same format. You can find them out in the MSDN document,And Microsoft named them as well-known SID.
SID: S-1-16-0
Name: Untrusted Mandatory Level
Description: An untrusted integrity level. Note Added in Windows Vista and Windows Server 2008
Note Added in Windows Vista and Windows Server 2008
SID: S-1-16-4096
Name: Low Mandatory Level
Description: A low integrity level.
Note Added in Windows Vista and Windows Server 2008
SID: S-1-16-8192
Name: Medium Mandatory Level
Description: A medium integrity level.
Note Added in Windows Vista and Windows Server 2008
SID: S-1-16-8448
Name: Medium Plus Mandatory Level
Description: A medium plus integrity level.
Note Added in Windows Vista and Windows Server 2008
SID: S-1-16-12288
Name: High Mandatory Level
Description: A high integrity level.
Note Added in Windows Vista and Windows Server 2008
SID: S-1-16-16384
Name: System Mandatory Level
Description: A system integrity level.
Note Added in Windows Vista and Windows Server 2008
SID: S-1-16-20480
Name: Protected Process Mandatory Level
Description: A protected-process integrity level.
Note Added in Windows Vista and Windows Server 2008
SID: S-1-16-28672
Name: Secure Process Mandatory Level
Description: A secure process integrity level.
Note Added in Windows Vista and Windows Server 2008
According to this, we known if the return value less than 0x1000 it indicate the security descripter's mandatory label is equal or less than Low Mandatory Level.
ProcessChildProcessPolicy
ProcessChildProcessPolicy has tree levels.
typedef struct _PROCESS_MITIGATION_CHILD_PROCESS_POLICY {
union {
DWORD Flags;
struct {
DWORD NoChildProcessCreation : 1;
DWORD AuditNoChildProcessCreation : 1;
DWORD AllowSecureProcessCreation : 1;
DWORD ReservedFlags : 29;
} DUMMYSTRUCTNAME;
} DUMMYUNIONNAME;
} PROCESS_MITIGATION_CHILD_PROCESS_POLICY, *PPROCESS_MITIGATION_CHILD_PROCESS_POLICY;
At the branch that handles ProcessChildProcessPolicy logic, it invokes a function named PspGetNoChildProcessRestrictedPolicy for getting the current process's child process mitigation policy status.
ChildPolicyLevel = PspGetNoChildProcessRestrictedPolicy(TargetProcess);
if(ChildPolicyLevel == 1)
{
PolicyInfo.NoChildProcessCreation = 1;
}
else if(ChildPolicyLevel == 2)
{
PolicyInfo.NoChildProcessCreation = 1;
PolicyInfo.AllowSecureProcessCreation = 1;
}
else if(ChildPolicyLevel == 3)
{
PolicyInfo.AuditNoChildProcessCreation = 1;
}
Unusually, it does not get the policy status from the EPROCESS's MitigationFlags field.In fact, it get some bit flags from the process token's TokenFlags field.The fake code look like this.
CurThread = KeGetCurrentThread();
TokenFlags = PrimaryToken->TokenFlags;
*NoChildProcessCreationFlag = (TokenFlags >> 19) & 1; // 20 bit
*AllowSecureProcessCreationFlag = (TokenFlags >> 20) & 1; // 21 bit
*AuditNoChildProcessCreationFlag = (TokenFlags >> 21) & 1; // 22 bit
There two ways to create a process.One is the NtCreateUserProcess function, and the other is the NtCreateProcess/NtCreateProcessEx.The NtCreateUserProcess is a newer native invoke that was introduced in Windows Vista. When we call CreateProcessW in user mode code, it will invoke NtCreateUserProcess function finally.
The ProcessChildProcessPolicy mitigation is implementation in the SeSubProcessToken.
1.NtCreateUserProcess
2.PspAllocateProcess
3.PspInitializeProcessSecurity
4.SeSubProcessToken
The SeSubProcessToken function is also invoke the PspGetNoChildProcessRestrictedPolicy to determine the the child can be created.If the creation desired is refused the NtCreateUserProcess will return the STATUS_CHILD_PROCESS_BLOCKED.
Sunday, October 27, 2019
Subscribe to:
Posts (Atom)
ACCESS_MASK: Specific rights of NT objects
#define FILE_READ_DATA ( 0x0001 ) // file & pipe #define FILE_LIST_DIRECTORY ( 0x0001 ) // directory #define FILE...
-
ProcessImageLoadPolicy In the recent years,Microsoft has add many mitigations in the Windows system. In this article i will show...
-
#define FILE_READ_DATA ( 0x0001 ) // file & pipe #define FILE_LIST_DIRECTORY ( 0x0001 ) // directory #define FILE...
-
Hello World!